A Simplified Information to Dynamic Programming
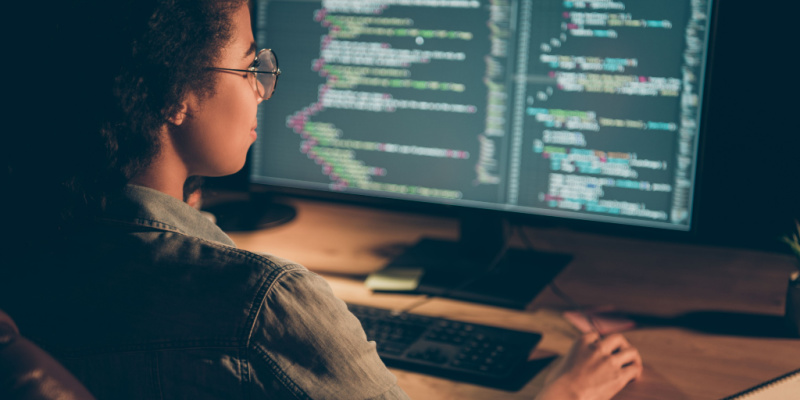
[ad_1]
Dynamic programming is outlined as a pc programming method the place an algorithmic downside is first damaged down into sub-problems, the outcomes are saved, after which the sub-problems are optimized to search out the general answer — which often has to do with discovering the utmost and minimal vary of the algorithmic question. This text intently examines how dynamic programming works, with examples.
What Is Dynamic Programming?
Dynamic programming is a pc programming method the place an algorithmic downside is first damaged down into sub-problems, the outcomes are saved, after which the sub-problems are optimized to search out the general answer — which often has to do with discovering the utmost and minimal vary of the algorithmic question.
Richard Bellman was the one who got here up with the concept for dynamic programming within the Nineteen Fifties. It’s a technique of mathematical optimization in addition to a technique for laptop programming. It applies to points one can break down into both overlapping subproblems or optimum substructures.
When a extra intensive set of equations is damaged down into smaller teams of equations, overlapping subproblems are known as equations that reuse parts of the smaller equations a number of occasions to reach at an answer.
However, optimum substructures find the most effective answer to a difficulty, then construct the answer that gives the most effective outcomes total. That is how they resolve issues. When an enormous subject is cut up down into its constituent elements, a pc will apply a mathematical algorithm to find out which parts have probably the most fascinating answer. Then, it takes the options to the extra minor issues and makes use of them to get the optimum answer to the preliminary, extra concerned subject.
This method solves issues by breaking them into smaller, overlapping subproblems. The outcomes are then saved in a desk to be reused so the identical downside won’t need to be computed once more.
For instance, when utilizing the dynamic programming method to determine all potential outcomes from a set of numbers, the primary time the outcomes are calculated, they’re saved and put into the equation later as an alternative of being calculated once more. So, when coping with lengthy, sophisticated equations and processes, it saves time and makes options quicker by doing much less work.
The dynamic programming algorithm tries to search out the shortest method to an answer when fixing an issue. It does this by going from the highest down or the underside up. The highest-down technique solves equations by breaking them into smaller ones and reusing the solutions when wanted. The underside-up strategy solves equations by breaking them up into smaller ones, then tries to unravel the equation with the smallest mathematical worth, after which works its method as much as the equation with the largest worth.
Utilizing dynamic programming to unravel issues is more practical than simply attempting issues till they work. But it surely solely helps with issues that one can break up into smaller equations that will likely be used once more in some unspecified time in the future.
See Extra: What Is Serverless? Definition, Structure, Examples, and Functions
Recursion vs. dynamic programming
In laptop science, recursion is a vital idea wherein the answer to an issue depends upon options to its smaller subproblems.
In the meantime, dynamic programming is an optimization method for recursive options. It’s the popular method for fixing recursive capabilities that make repeated calls to the identical inputs. A operate is named recursive if it calls itself throughout execution. This course of can repeat itself a number of occasions earlier than the answer is computed and may repeat without end if it lacks a base case to allow it to satisfy its computation and cease the execution.
Nonetheless, not all issues that use recursion may be solved by dynamic programming. Until options to the subproblems overlap, a recursion answer can solely be arrived at utilizing a divide-and-conquer technique.
For instance, issues like merge, kind, and fast kind should not thought-about dynamic programming issues. It’s because they contain placing collectively the most effective solutions to subproblems that don’t overlap.
Drawbacks of recursion
Recursion makes use of reminiscence house much less effectively. Repeated operate calls create entries for all of the variables and constants within the operate stack. Because the values are saved there till the operate returns, there may be at all times a restricted quantity of stack house within the system, thus making much less environment friendly use of reminiscence house. Moreover, a stack overflow error happens if the recursive operate requires extra reminiscence than is obtainable within the stack.
Recursion can be comparatively sluggish compared to iteration, which makes use of loops. When a operate is named, there may be an overhead of allocating house for the operate and all its knowledge within the operate stack in recursion. This causes a slight delay in recursive capabilities.
The place ought to dynamic programming be used?
Dynamic programming is used when one can break an issue into extra minor points that they’ll break down even additional, into much more minor issues. Moreover, these subproblems have overlapped. That’s, they require beforehand calculated values to be recomputed. With dynamic programming, the computed values are saved, thus decreasing the necessity for repeated calculations and saving time and offering quicker options.
How Does Dynamic Programming Work?
Dynamic programming works by breaking down advanced issues into less complicated subproblems. Then, discovering optimum options to those subproblems. Memorization is a technique that saves the outcomes of those processes in order that the corresponding solutions don’t should be computed when they’re later wanted. Saving options save time on the computation of subproblems which have already been encountered.
Dynamic programming may be achieved utilizing two approaches:
1. High-down strategy
In laptop science, issues are resolved by recursively formulating options, using the solutions to the issues’ subproblems. If the solutions to the subproblems overlap, they could be memoized or saved in a desk for later use. The highest-down strategy follows the technique of memorization. The memoization course of is equal to including the recursion and caching steps. The distinction between recursion and caching is that recursion requires calling the operate immediately, whereas caching requires preserving the intermediate outcomes.
The highest-down technique has many advantages, together with the next:
- The highest-down strategy is simple to grasp and implement. On this strategy, issues are damaged down into smaller elements, which assist customers determine what must be completed. With every step, extra important, extra advanced issues turn out to be smaller, easier, and, subsequently, simpler to unravel. Some elements might even be reusable for a similar downside.
- It permits for subproblems to be solved upon request. The highest-down strategy will allow issues to be damaged down into smaller elements and their options saved for reuse. Customers can then question options for every half.
- It is usually simpler to debug. Segmenting issues into small elements permits customers to comply with the answer shortly and decide the place an error might need occurred.
Disadvantages of the top-down strategy embody:
- The highest-down strategy makes use of the recursion method, which occupies extra reminiscence within the name stack. This results in diminished total efficiency. Moreover, when the recursion is simply too deep, a stack overflow happens.
2. Backside-up strategy
Within the bottom-up technique, as soon as an answer to an issue is written by way of its subproblems in a method that loops again on itself, customers can rewrite the issue by fixing the smaller subproblems first after which utilizing their options to unravel the bigger subproblems.
In contrast to the top-down strategy, the bottom-up strategy removes the recursion. Thus, there may be neither stack overflow nor overhead from the recursive capabilities. It additionally permits for saving reminiscence house. Eradicating recursion decreases the time complexity of recursion as a result of recalculating the identical values.
The benefits of the bottom-up strategy embody the next:
- It makes selections about small reusable subproblems after which decides how they are going to be put collectively to create a big downside.
- It removes recursion, thus selling the environment friendly use of reminiscence house. Moreover, this additionally results in a discount in timing complexity.
See Extra: DevOps Roadmap: 7-Step Full Information
Indicators of dynamic programming suitability
Dynamic programming solves advanced issues by breaking them up into smaller ones utilizing recursion and storing the solutions so that they don’t need to be labored out once more. It isn’t sensible when there aren’t any issues that overlap as a result of it doesn’t make sense to retailer options to the problems that received’t be wanted once more.
Two major indicators are that one can resolve an issue with dynamic programming: subproblems that overlap and the very best substructure.
Overlapping subproblems
When the solutions to the identical subproblem are wanted greater than as soon as to unravel the primary downside, we are saying that the subproblems overlap. In overlapping points, options are put right into a desk so builders can use them repeatedly as an alternative of recalculating them. The recursive program for the Fibonacci numbers has a number of subproblems that overlap, however a binary search doesn’t have any subproblems that overlap.
A binary search is solved utilizing the divide and conquer method. Each time, the subproblems have a novel array to search out the worth. Thus, binary search lacks the overlapping property.
For instance, when discovering the nth Fibonacci quantity, the issue F(n) is damaged down into discovering F(n-1) and F. (n-2). You may break down F(n-1) even additional right into a subproblem that has to do with F. (n-2).On this state of affairs, F(n-2) is reused, and thus, the Fibonacci sequence may be stated to exhibit overlapping properties.
Optimum substructure
The optimum substructure property of an issue says that you could find the most effective reply to the issue by taking the most effective options to its subproblems and placing them collectively. More often than not, recursion explains how these optimum substructures work.
This property will not be unique to dynamic programming alone, as a number of issues encompass optimum substructures. Nonetheless, most of them lack overlapping points. So, they’ll’t be known as issues with dynamic programming.
You should utilize it to search out the shortest route between two factors. For instance, if a node p is on the shortest path from a supply node t to a vacation spot node w, then the shortest path from t to w is the sum of the shortest paths from t to p and from p to w.
Examples of issues with optimum substructures embody the longest rising subsequence, longest palindromic substring, and longest widespread subsequence downside. Examples of issues with out optimum substructures embody probably the most prolonged path downside and the addition-chain exponentiation.
Understanding the Longest Frequent Subsequence idea in dynamic programming
In dynamic programming, the phrase “largest widespread subsequence” (LCS) refers back to the subsequence that’s shared by the entire provided sequences and is the one that’s the longest. It’s totally different from the problem of discovering the longest widespread substring in that the elements of the LCS don’t must occupy consecutive places inside the unique sequences to be thought-about a part of that downside.
The LCS is characterised by an optimum substructure and overlapping subproblem properties. This means that the difficulty could also be cut up into many much less advanced sub-issues and labored on individually till an answer is discovered. The options to higher-level subproblems are sometimes reused in lower-level subproblems, thus, overlapping subproblems.
Subsequently, when fixing an LCS downside, it’s extra environment friendly to make use of a dynamic algorithm than a recursive algorithm. Dynamic programming shops the outcomes of every operate name in order that it may be utilized in future calls, thus minimizing the necessity for redundant calls.
As an example, think about the sequences (MNOP) and (MONMP). They’ve 5 length-2 widespread subsequences (MN), (MO), (MP), (NP), and (OP); two length-3 widespread subsequences (MNP) and (MOP); MNP and not frequent subsequences (MOP). Consequently, (MNP) and (MOP) are the most important shared subsequences. LCS may be utilized in bioinformatics to the method of genome sequencing.
See Extra: What Is Jenkins? Working, Makes use of, Pipelines, and Options
Dynamic Programming Algorithms
When dynamic programming algorithms are executed, they resolve an issue by segmenting it into smaller elements till an answer arrives. They carry out these duties by discovering the shortest path. A number of the main dynamic programming algorithms in use are:
1. Grasping algorithms
An instance of dynamic programming algorithms, grasping algorithms are additionally optimization instruments. The tactic solves a problem by looking for optimum options to the subproblems and mixing the findings of those subproblems to get probably the most optimum reply.
Conversely, when grasping algorithms resolve an issue, they search for a domestically optimum answer to discover a world optimum. They make a guess that appears optimum on the time however doesn’t assure a globally optimum answer. This might find yourself turning into expensive down the street.
2. Floyd-Warshall algorithm
The Floyd-Warshall technique makes use of a way of dynamic programming to find the shortest pathways. It determines the shortest route throughout all pairings of vertices in a graph with weights. Each directed and undirected weighted graphs can use it.
This program compares every pair of vertices’ potential routes by way of the graph. It progressively optimizes an estimate of the shortest route between two vertices to find out the shortest distance between two vertices in a chart. With easy modifications to it, one can reconstruct the paths.
This technique for dynamic programming comprises two subtypes:
- Conduct with unfavourable cycles: Customers can use the Floyd-Warshall algorithm to search out unfavourable cycles. You are able to do this by inspecting the diagonal path matrix for a unfavourable quantity that will point out the graph comprises one unfavourable cycle. In a unfavourable cycle, the sum of the perimeters is a unfavourable worth; thus, there can’t be a shortest path between any pair of vertices. Exponentially enormous numbers are generated if a unfavourable cycle happens throughout algorithm execution.
- Time complexity: The Floyd-Warshall algorithm has three loops, every with fixed complexity. In consequence, the Floyd-Warshall complexity has a time complexity of O(n3). Whereby n represents the variety of community nodes.
3. Bellman Ford algorithm
The Bellman-Ford Algorithm determines the shortest route from a specific supply vertex to each different weighted digraph vertices. The Bellman-Ford algorithm can deal with graphs the place among the edge weights are unfavourable numbers and produce an accurate reply, in contrast to Dijkstra’s algorithm, which doesn’t verify whether or not it makes the right reply. Nonetheless, it’s a lot slower than Dijkstra’s algorithm.
The Bellman-Ford algorithm works by rest; that’s, it provides approximate distances that higher ones repeatedly change till an answer is reached. The approximate distances are often overestimated in comparison with the gap between the vertices. The alternative values mirror the minimal previous worth and the size of a newly discovered path.
This algorithm terminates upon discovering a unfavourable cycle and thus may be utilized to cycle-canceling strategies in community move evaluation.
See Extra: High 10 DevOps Automation Instruments in 2021
Examples of Dynamic Programming
Listed below are just a few examples of how one might use dynamic programming:
1. Figuring out the variety of methods to cowl a distance
Some recursive capabilities are invoked 3 times within the recursion method, indicating the overlapping subproblem attribute required to calculate points that use the dynamic programming methodology.
Utilizing the top-down method, simply retailer the worth in a HashMap whereas retaining the recursive construction, then return the worth retailer with out calculating every time the operate is invoked. Make the most of an additional house of dimension n when using the bottom-up technique and compute the values of states starting with 1, 2,…, n, i.e., compute the values of I i+1 and that i+2 after which apply them to find out the worth of i+3.
2. Figuring out the optimum technique of a sport
To determine the optimum technique of a sport or gamified expertise, let’s think about the “cash in a line” sport. The memoization method is used to compute the utmost worth of cash taken by participant A for cash numbered h to ok, assuming participant B performs optimally (Mh,ok). To seek out out every participant’s technique, assign values to the coin they choose and the worth of the opponent’s coin. After computation, the optimum design for the sport is set by observing the Mh,ok worth for each gamers if participant A chooses coin h or ok.
3. Counting the variety of potential outcomes of a specific die roll
With an integer M, the purpose is to find out the variety of approaches to acquire the sum M by tossing cube repeatedly. The partial recursion tree, the place M=8, supplies overlapping subproblems when utilizing the recursion technique. Through the use of dynamic programming, one can optimize the recursive technique. One can use an array to retailer values after computation for reuse. On this method, the algorithm takes considerably much less time to run with time advanced: O(t * n * m), with t being the variety of faces, n being the variety of cube, and m being the given sum.
See Extra: DevOps vs. Agile Methodology: Key Variations and Similarities
Takeaway
Dynamic programming is among the many extra superior expertise one should be taught as a programmer or DevOps engineer, primarily in the event you concentrate on Python. It’s a comparatively easy method to resolve advanced algorithmic issues and a ability you’ll be able to apply to nearly any language or use case. For instance, the viral sport, Wordle, follows dynamic programming rules, and customers can prepare an algorithm to resolve it by discovering probably the most optimum mixture of alphabets. In different phrases, the ability has versatile functions and should be a part of each DevOps studying package.
Did this text provide help to perceive how dynamic programming works? Inform us on Fb, Twitter, and LinkedIn. We’d love to listen to from you!
MORE ON DEVOPS
[ad_2]
Source_link