7 Database Optimization Finest Practices for Django Builders
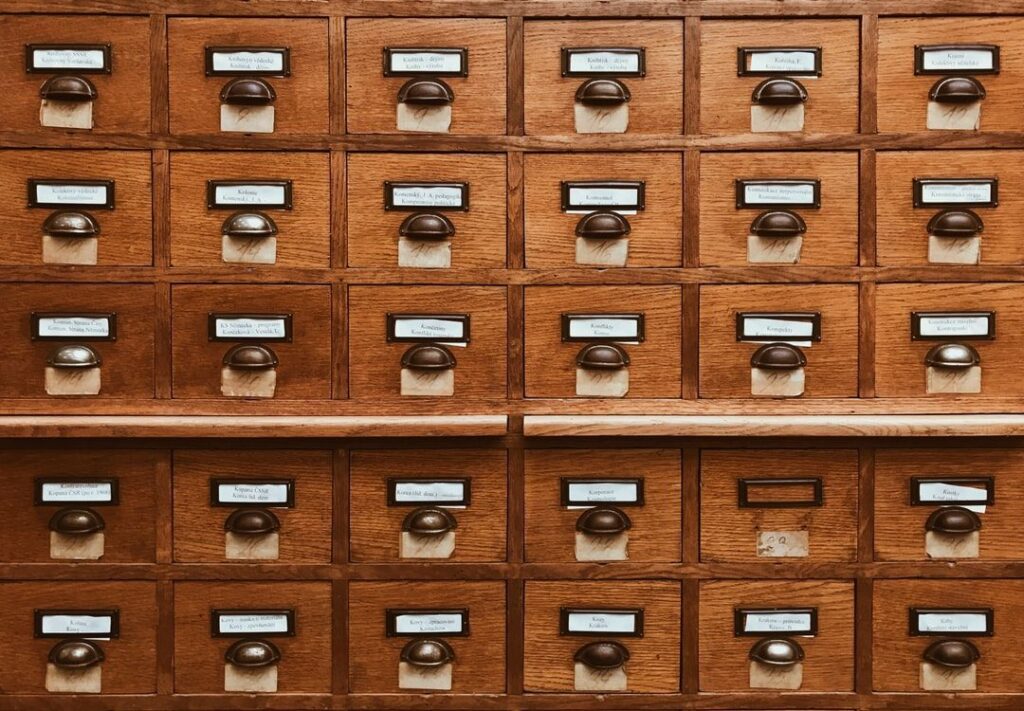
[ad_1]
Database administration is without doubt one of the most vital features of backend improvement. A correctly optimized database can assist to scale back the response time and therefore result in a greater person expertise.
On this article, we will likely be discussing the methods to optimize the database for velocity in Django functions. Though, we gained’t be diving deep into every idea individually, therefore, please seek advice from the official Django documentation for full particulars.
Understanding Queries in Django
Understanding querysets in Django is the important thing to optimization, therefore, please bear in mind the next:
- Querysets are lazy, which signifies that no corresponding database request is made till you carry out sure actions on the queryset, equivalent to iterating over it.
- At all times restrict the results of a database question by specifying the variety of values to be returned.
- In Django, querysets might be evaluated by iteration, slicing, caching, and python strategies equivalent to
len()
,rely()
and so on. Just be sure you make the most effective use of them. - Django querysets are cached, such that in the event you re-use the identical queryset, a number of database requests gained’t be made, thus minimizing database entry.
- Retrieve every little thing that you’d want directly, however guarantee that you’re retrieving solely what you want.
Question Optimization in Django
Database Indexing
Database indexing is a way for rushing up queries whereas retrieving information from a database. As the applying will increase in dimension, it could decelerate, and customers will discover since it is going to take considerably longer to acquire the required information. Thus, indexing is a non-negotiable operation when working with giant databases that generate an enormous quantity of information.
Indexing is a technique of sorting numerous information primarily based on numerous fields. While you create an index on a discipline in a database, you create one other information construction that comprises the sphere worth in addition to a pointer to the report to which it’s associated. This index construction is then sorted, making Binary Searches attainable.
For instance, here’s a Django mannequin named Sale:
# fashions.py
from django.db import fashions
class Sale(fashions.Mannequin):
sold_at = fashions.DateTimeField(
auto_now_add=True,
)
charged_amount = fashions.PositiveIntegerField()
Database Indexing might be added to a selected discipline whereas defining a Django mannequin as follows:
# fashions.py
from django.db import fashions
class Sale(fashions.Mannequin):
sold_at = fashions.DateTimeField(
auto_now_add=True,
db_index=True, #DB Indexing
)
charged_amount = fashions.PositiveIntegerField()
If you happen to run the migrations for this mannequin, Django will create a database index on the desk Gross sales, and it is going to be locked till the index is accomplished. On a neighborhood improvement setup, with a small quantity of information and only a few connections, this migration would possibly really feel instantaneous, however once we speak concerning the manufacturing setting, there are giant datasets with many concurrent connections that may trigger downtime as acquiring a lock and making a database index can take plenty of time.
You can even create a single index for 2 fields as proven beneath:
# fashions.py
from django.db import fashions
class Sale(fashions.Mannequin):
sold_at = fashions.DateTimeField(
auto_now_add=True,
db_index=True, #DB Indexing
)
charged_amount = fashions.PositiveIntegerField()
class Meta:
indexes = [
["sold_at", "charged_amount"]]
Database Caching
Database caching is without doubt one of the finest approaches to getting a quick response from a database. It ensures that fewer calls are made to the database, stopping overload. A normal caching operation follows the beneath construction:
Django gives a caching mechanism that may use completely different caching backends like Memcached and Redis that allow you to keep away from operating the identical queries a number of occasions.
Memcached is an open-source in-memory system that ensures to supply cached ends in lower than a millisecond. It’s easy to arrange and scale. Redis, alternatively, is an open-source caching resolution with related traits to Memcached. Most offline apps make use of beforehand cached information, which means that almost all of queries by no means ever attain the database.
Person classes must be saved in a cache in your Django software, and since Redis maintains information on disk, all classes for logged-in customers originate from the cache relatively than the database.
To make use of Memcache with Django, we have to outline the next:
- BACKEND: To outline the cache backend to make use of.
- LOCATION:
ip:port
values the placeip
is the IP deal with of the Memcached daemon andport
is the port on which Memcached is operating, or the URL pointing to your Redis occasion, utilizing the suitable scheme.
To allow database caching with Memcached, set up pymemcache
utilizing pip utilizing the next command:
pip set up pymemcache
Then, you’ll be able to configure the cache settings in your settings.py
as follows:
CACHES = {
'default': {
'BACKEND': 'django.core.cache.backends.memcached.PyMemcacheCache',
'LOCATION': '127.0.0.1:11211',
}
}
Within the above instance, Memcached is operating on localhost (127.0.0.1) port 11211, utilizing the pymemcache
binding:
Equally, to allow database caching utilizing Redis, set up Redis utilizing pip utilizing the command beneath:
pip set up redis
Then configure the cache settings in your settings.py
by including the next code:
CACHES = {
'default': {
'BACKEND': 'django.core.cache.backends.redis.RedisCache',
'LOCATION': 'redis://127.0.0.1:6379',
}
}
Memcached and Redis may also be used to retailer person authentication tokens. As a result of each one who logs in should provide a token, all of those procedures may end up in important database overhead. Utilizing cached tokens will end in significantly faster database entry.
Utilizing Iterator When Potential
A queryset in Django, sometimes, caches its end result when analysis occurs and for any additional operation with that queryset, it first checks if there are cached outcomes. Nonetheless, once you use iterator()
, it doesn’t examine for cache and reads outcomes straight from the database, neither saves the outcomes to the queryset.
Now, you should be questioning how that is useful. Contemplate a queryset that returns numerous objects with plenty of reminiscence to cache however must be used solely as soon as, in such case, you must use an iterator()
.
As an illustration, within the following code, all information will likely be fetched from the database after which loaded within the reminiscence after which we’ll iterate by every one:
queryset = Product.objects.all()
for every in queryset:
do_something(every)
Whereas if we use iterator()
, Django will maintain the SQL connection open and skim every report, and name do_something()
earlier than studying the subsequent report:
queryset = Product.objects.all().iterator()
for every in queryset:
do_something(every)
Utilizing a Persistence Database Connection
Django creates a brand new database connection for every request and closes it after the request is full. This conduct is brought on by CONN_MAX_AGE
, which has a default worth of 0. However how lengthy ought to or not it’s set to? That’s decided by the quantity of site visitors in your website; the upper the quantity, the extra seconds are required to keep up the connection. It’s often advisable to start out with a low quantity, equivalent to 60.
It’s essential wrap your additional choices in OPTIONS
, as detailed within the
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.mysql',
'NAME': 'dashboard',
'USER': 'root',
'PASSWORD': 'root',
'HOST': '127.0.0.1',
'PORT': '3306',
'OPTIONS': {
'CONN_MAX_AGE': '60',
}
}
}
Utilizing Question Expressions
Question expressions outline a price or a computation that could be utilized in an replace, create, filter, order by, annotation, or mixture operation.
A generally used built-in question expression in Django is the F expression. Let’s see the way it works and might be helpful.
Word: These expressions are outlined in django.db.fashions.expressions
and django.db.fashions.aggregates
, however for comfort, they’re accessible and often imported from django.db.fashions
.
F Expression
Within the Django Queryset API, F()
expressions are used to seek advice from the mannequin discipline values straight. It permits you to seek advice from mannequin discipline values and conduct database actions on them with out having to fetch them from the database and into Python reminiscence. As a substitute, Django employs the F()
object to provide a SQL phrase that defines the wanted database exercise.
For instance, let’s say that we wish to improve the value of all merchandise by 20%, then the code would look one thing like this:
merchandise = Product.objects.all()
for product in merchandise:
product.worth *= 1.2
product.save()
Nonetheless, if we use F()
, we are able to do that in a single question as follows:
from django.db.fashions import F
Product.objects.replace(worth=F('worth') * 1.2)
Utilizing select_related()
and prefetch_related()
Django gives select_related()
and prefetch_related()
arguments for optimizing your querysets by minimizing the variety of database requests.
In line with the official Django Documentation:
select_related()
“follows” foreign-key relationships, choosing extra related-object information when it executes its question.
prefetch_related()
does a separate lookup for every relationship, and does the “becoming a member of” in Python.
select_related()
We use select_related()
when the merchandise to be chosen is a single object which implies ahead ForeignKey
, OneToOne
, and backward OneToOne
discipline.
Chances are you’ll use select_related()
to create a single question that returns all of the associated objects for a single occasion for one-many and one-to-one connections. When the question is carried out, select_related()
retrieves any additional related-object information from foreign-key relationships.
select_related()
works by producing a SQL be a part of and contains the associated object’s columns within the SELECT
expression. Consequently, select_related()
returns associated gadgets in the identical database question.
Although select_related()
produces a extra subtle question, the info acquired is cached, thus dealing with of the info obtained doesn’t necessitate any additional database requests.
The syntax merely appears like this:
queryset = Tweet.objects.select_related('proprietor').all()
prefetch_related()
In distinction, prefetch_related()
is utilized for many-to-many and many-to-one connections. It produces a single question that features all the fashions and filters are given within the question.
The syntax merely appears like this:
E book.objects.prefetch_related('creator').get(id=1).creator.first_name
NOTE: ManyToMany relationships shouldn’t be dealt with utilizing SQL as a result of many efficiency points might seem when coping with giant tables. That is why the prefetch_related methodology joins tables inside Python avoiding making giant SQL joins.
Learn concerning the distinction between select_related()
and prefetch_related()
intimately right here.
Utilizing bulk_create()
and bulk_update()
bulk_create()
is a technique that creates the offered record of objects into the database with one question. Equally, bulk_update()
is a technique that updates the given fields on the offered mannequin cases with one question.
For instance, if we have now a posts mannequin as proven beneath:
class Put up(fashions.Mannequin):
title = fashions.CharField(max_length=300, distinctive=True)
time = fashions.DateTimeField(auto_now_add=True)
def __str__(self):
return self.title
Now, let’s say that we wish to add a number of information information to this mannequin, then we are able to use bulk_create()
like this:
#articles
articles = [Post(title="Hello python"), Post(title="Hello django"), Post(title="Hello bulk")]
#insert information
Put up.objects.bulk_create(articles)
And the output would appear like this:
>>> Put up.objects.all()
<QuerySet [<Post: Hello python>, <Post: Hello django>, <Post: Hello bulk>]>
And if we wish to replace the info, then we are able to use bulk_update()
like this:
update_queries = []
a = Put up.objects.get(id=14)
b = Put up.objects.get(id=15)
c = Put up.objects.get(id=16)
#set replace worth
a.title="Howdy python up to date"
b.title="Howdy django up to date"
c.title="Howdy bulk up to date"
#append
update_queries.lengthen((a, b, c))
Put up.objects.bulk_update(update_queries, ['title'])
And the output would appear like this:
>>> Put up.objects.all()
<QuerySet [<Post: Hello python updated>, <Post: Hello django updated>, <Post: Hello bulk updated>]>
Conclusion
On this article, we coated the tricks to optimize the efficiency of the database, scale back bottlenecks and save sources in a Django software.
I hope you discovered it useful. Maintain studying!
L O A D I N G
. . . feedback & extra!
[ad_2]
Source_link