Fixing an Optimization Drawback in R Utilizing Linear Programming | by Andrew Josselyn | Dec, 2022
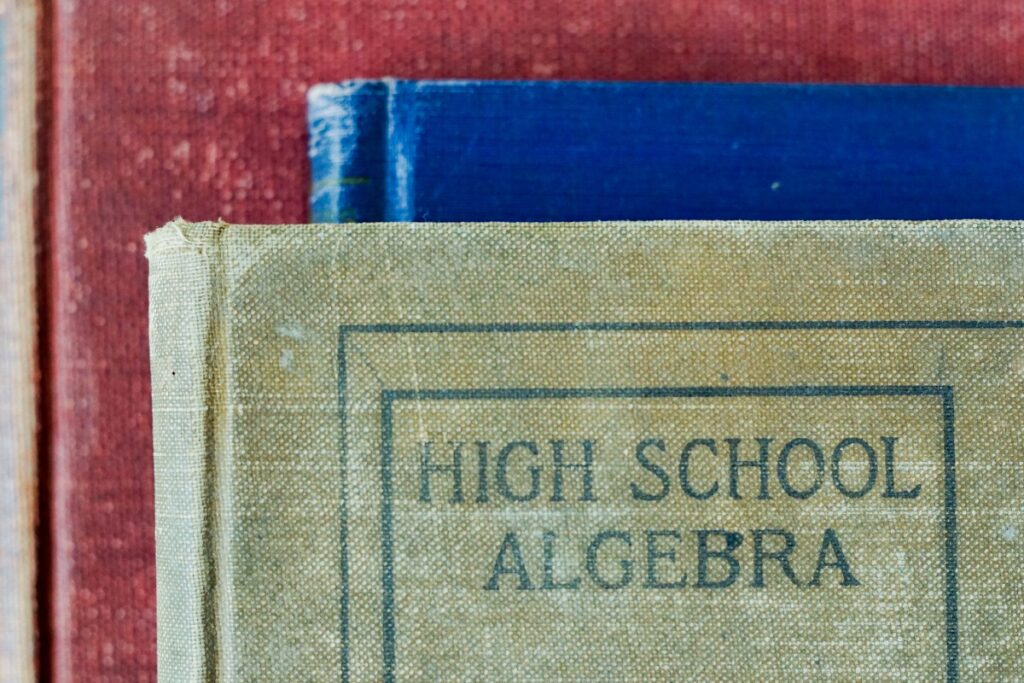
[ad_1]
Time to brush up on our Linear Algebra expertise.
Optimization strategies are extraordinarily highly effective instruments for guaranteeing the perfect selections are made. Nonetheless, a variety of Information Analysts and Information Scientists enter the workforce with none expertise implementing these expertise to drive higher enterprise selections. For myself, two expertise I wanted to be taught rapidly as a Information Scientist had been time sequence evaluation and optimization. On this evaluation I’ll use R-Studio to resolve a linear optimization drawback.
The issue I’ll use comes from Joel Sobel’s, a Professor of Economics at UC San Diego, Econ 172B notes discovered right here: https://econweb.ucsd.edu/~jsobel/172aw02/notes8.pdf
A lumber firm has three sources of wooden and 5 markets the place wooden is demanded. The annual amount of wooden accessible within the three sources of provide are 15, 20, and 15 million board toes respectively. The quantity that may be offered on the 5 markets is 11, 12, 9, 10, and eight million board toes, respectively. The corporate at the moment transports the entire wooden by practice. It needs to guage its transportation schedule, probably shifting some or all of its transportation to ships. The unit price of cargo (in $10,000) alongside the assorted routes utilizing each strategies is described within the desk beneath.
The administration must resolve to what extent to proceed to depend on rail transportation. Consider the next choices and make a suggestion about what to do.
- How a lot does it price to make use of rail transport solely?
- How a lot does it price to make use of ships solely?
- How a lot does it price to make use of the most cost effective accessible mode of transportation on every route?
- Suppose that there’s an annual price of $100,000 to function any ships (however that this price doesn’t range with the variety of transport traces saved open). What’s the optimum transportation plan?
Studying this drawback gave me an immediate panic assault and made me really feel like I used to be writing an undergrad examination once more. Nonetheless, if we break the issue up into small components, it’s not as daunting because it seems. Linear Programming seeks to seek out the values of the resolution variables that optimize (maximize or decrease) an goal perform among the many set of all values for the choice variables that fulfill the given constraints.
Query 1: How a lot does it price to make use of rail transport solely?
### Set up and Load Libraries ###
set up.packages('lpSolve')
library(lpSolve)### Query 1: Rail Solely ###
# Write the Goal Perform
Rail_obj <- c(51, 62, 35, 45, 56, 59, 68, 50, 39, 46, 49, 56, 53, 51, 37)
# Set matrix akin to coefficients of constraints by rows
Rail_con <- matrix(c(1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0,
0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0,
0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0,
0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0,
0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1,
1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1
), nrow = 8, byrow = TRUE)
# Set unequality indicators
Rail_dir <- c("<=",
"<=",
"<=",
"<=",
"<=",
"=",
"=",
"=")
# Set proper hand aspect coefficients
Rail_rhs <- c(11,
12,
9,
10,
8,
15,
20,
15
)
# Variables closing values
lp("min", Rail_obj, Rail_con, Rail_dir, Rail_rhs)$resolution
Operating this code will give us an optimized (minimal price) resolution of:
This ends in a complete price of $23,160,000 for under transport with rail.
Query 2: How a lot does it price to make use of ships solely?
That is an attention-grabbing drawback as a result of we’ve two situations during which transport isn’t an choice (“none”). To beat this constraint, as a result of we are attempting to reduce prices, we are able to set these values to a big quantity:
### Query 2: Transport Solely ###
# Write the Goal Perform, change "none" with a big quantity
Ship_obj <- c(48, 68, 48, 999, 54, 66, 75, 55, 49, 57, 999, 61, 64, 59, 50)# Set matrix akin to coefficients of constraints by rows
Ship_con <- matrix(c(1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0,
0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0,
0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0,
0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0,
0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1,
1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1
), nrow = 8, byrow = TRUE)
# Set unequality indicators
Ship_dir <- c("<=",
"<=",
"<=",
"<=",
"<=",
"=",
"=",
"=")
# Set proper hand aspect coefficients
Ship_rhs <- c(11,
12,
9,
10,
8,
15,
20,
15
)
# Variables closing values
lp("min", Ship_obj, Ship_con, Ship_dir, Ship_rhs)$resolution
This code provides us the minimal transport price resolution of:
The prices of solely utilizing ships is $26,520,000, which is $3,360,000 greater than utilizing simply rail ($23,160,000).
Query 3: How a lot does it price to make use of the most cost effective accessible mode of transportation on every route?
To resolve this drawback we are going to put the smallest price for every market and provide into our goal perform.
### Query 3: Optimum ###
# Write the Goal Perform
optimal_obj <- c(48, 62, 35, 45, 54, 59, 68, 50, 39, 46, 49, 56, 53, 51, 37)# Set matrix akin to coefficients of constraints by rows
optimal_con <- matrix(c(1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0,
0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0,
0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0,
0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0,
0, 0, 0, 0, 1, 0, 0, 0, 0, 1, 0, 0, 0, 0, 1,
1, 1, 1, 1, 1, 0, 0, 0, 0, 0, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 1, 1, 1, 1, 1, 0, 0, 0, 0, 0,
0, 0, 0, 0, 0, 0, 0, 0, 0, 0, 1, 1, 1, 1, 1
), nrow = 8, byrow = TRUE)
# Set unequality indicators
optimal_dir <- c("<=",
"<=",
"<=",
"<=",
"<=",
"=",
"=",
"=")
# Set proper hand aspect coefficients
optimal_rhs <- c(11,
12,
9,
10,
8,
15,
20,
15
)
# Variables closing values
lp("min", optimal_obj, optimal_con, optimal_dir, optimal_rhs)$resolution
The optimum transport is:
This transport ends in an optimum transport price of $22,980,000, which is $180,000 much less than simply utilizing rail to ship ($23,160,000).
Query 4: Suppose that there’s an annual price of $100,000 to function any ships (however that this price doesn’t range with the variety of transport traces saved open). What’s the optimum transportation plan?
From our outcomes above:
Optimally transport with each rail and ships is $180,000 inexpensive than transport with simply rail and $3,540,000 inexpensive than transport with simply ships. Even when we needed to pay a further $100,000 to make use of ships it might be value it and permit us to save lots of $80,000 per 12 months on decreased transport prices.
On this evaluation I’ve proven that utilizing Linear Programming can present huge insights when making enterprise selections. Using Linear Programming took a really advanced drawback and was capable of simplify it to offer concrete insights on what to do. On this case, paying $100,000 to permit shipments of Provide A lumber to be made to Market 1 by ships, will end in $80,000 of financial savings per 12 months.
[ad_2]
Source_link